Documentation
This page explains how I modified the original documentation page to make it look like this. Here is a cheat sheet I like, which summarizes the main syntax elements of markdown.
- As a developper, using git and GitLab is something I do on a day-to-day basis. Here's a list of tutorials for git
- I'm using Linux and the command-line to do most things, because it is how I usually do things
- Knowledge of HTML and CSS is probably necessary to follow this. Here is a basic tutorial
Working on the project locally
The first thing I did was cloning the project on my computer, to be able to work on it in my code editor. Here is how I cloned the project on my machine and installed the requirements inside a virtual environment:
$ mkdir fabricademy
$ cd fabricademy
$ git clone git@gitlab.fabcloud.org:academany/fabricademy/2021/students/diane.wakim.git
$ virtualenv fabenv
$ source fabenv/bin/activate
$ cd diane.wakim
$ pip install requirements.txt
What's happening here, line by line:
- Creating a folder named
fabricademy
; - Going inside that folder;
- Cloning the repository from gitlab. This will create a folder named
diane.wakim
, which is the name of the repo; - Creating a virtual environment (this assumes virtualenv is already installed). This is important so that there is no interference when different code libraries are installed, so that they do not clash with maybe another version of it that you already have...
- Activating the virtual environment;
- Going inside the git repo that was create when we cloned it;
- Installing the requirements for the repo. These are mainly the site generator, MkDocs, and the visual theme for the project. It's important to install MkDocs using the requirements file and not with your usual package manager, because the version used here is not the last existing version.
After adding content, images or editing anything, I commit the changes, and push them to the repository:
$ git add .
$ git commit -m "A nice commit message"
$ git push origin master
I then usually got to check on gitlab if the process is executing correctly.
Editing the theme
I did not want to completely change the website and replace it with something else, because I quite like static site generators. So I made it an excercise to see how far I could go by not changing the theme, but only tweaking it.
Visually, I like simple websites, but here I still need a bit of structure for the documentation, so I kept most of the existing structure. You can see the whole code here, but here is a list of the things I changed:
Overriding templates
I wanted to have a different kind of menu on top of the site, so I wanted to change the header template. In order to change templates from the theme, we can override them by creating a same-named file in a specific place: the overrides
folder. This folder should be located at the root of the repository. In it, should be only files from the theme that you want to override.
We are using the theme "Material", and the files we want to override need to be in the exact same place as they are in the source theme. I checked the theme's source in its repository to mimick the structure, and copy the content of the files I needed before modifying them.
The header file, for example, is located in a folder named "partials". Here is the structure of my override directory (which you can also see on gitlab), with all the files I changed:
> overrides/
> main.html
> partials/
> header.html
> tabs.html
> toc.html
Some of them don't have strong modifications. In toc.html
, which is the template for the table of contents on the right of each page, I just changed the title to write "On this page" instead of the title of the page, because I found it redundant.
The file tabs.html is used for the dropdowns of the header menu; I did not want to use them, so the file overriding this is empty.
Using HTML in Markdown files
I you find yourself limited by what you can do with Markdown, you can write HTML directly in the markdown page. It will stay as-is in the final page, the compiler won't need to "translate" it. I mainly used this for 2 reasons:
- Having some links open in a new tab.
There is now way to do this in simple Markdown. In HTML we just need to add the target
attribute to the link tag:
<a href="/" target="_blank">My Homepage</a>
This code gives us the following link: My Homepage.
- Having stand-out sections that I could add a CSS class to.
I like to have some sections where I can write things differently. You can see some on this page, for example the "disclaimers" on top of the page. Here is the code for this specific one:
<div class="callout">
<span class="callout-emoji">⚠️</span>
<div>
<span class="highlight">A few disclaimers:</span>
<ul>
<li>As a developper, using <a href="https://git-scm.com/" target="_blank">git</a> and GitLab is something I do on a day-to-day basis. Here's a <a href="https://try.github.io/" target="_blank">list of tutorials</a> for git</li>
<li>I'm using Linux and the command-line to do most things, because it is how I usually do things</li>
<li>Knowledge of HTML and CSS is probably necessary to follow this. Here is a <a href="https://html.com/" target="_blank">basic tutorial</a></li>
</ul>
</div>
</div>
I always use this same structure, with the main tag having the callout
CSS class. I then use this class to define the styles and colors of these sections.
Note that if you add HTML, you cannot write Markdown inside of it. For example in the stand-out sections I describe above, once I'm inside the <div>
tag, everything had to be HTML.
Overriding CSS
I added some custom CSS, in docs/stylesheets/custom.css
. This file is then referenced in the config file mkdocs.yml
by the following lines:
extra_css:
- 'stylesheets/custom.css'
Adding Javascript
I added some extra behaviour with Javascript. The first function is here mainly because I modified the header in kind of a harsh way, and I wanted to add something that would bring back mobile responsiveness for the header menu. It hides and shows the dropdown menu when viewing the site from a mobile phone.
// mobile dropdown menu
let menuList = document.querySelector(".md-tabs__list");
let openMenuLink = document.querySelector(".mobile-menu a.open-menu");
let closeMenuLink = document.querySelector(".mobile-menu a.close-menu");
closeMenuLink.addEventListener('click', function(event){
menuList.classList.remove("mobile");
openMenuLink.classList.remove("hidden");
closeMenuLink.classList.add("hidden");
});
openMenuLink.addEventListener('click', function(event){
menuList.classList.add("mobile");
openMenuLink.classList.add("hidden");
closeMenuLink.classList.remove("hidden");
});
This second function is me pushing the theme to its limits... This theme is not supposed to have sections page, that is to say when you click on "Assignments", there is no global Assignments page, you will directly go to the first assignment in the list (week 1). I really wanted sections pages, to have nice view of all the assignments, so I did the following things:
- create an index.md in each folder (assignments, project, etc.)
- have this function redirect to this index.md page when clicking on one of the section in the menu
Clearly this theme is not made for this, and this is a bit of an ugly hack, but it works for now 🤷🏻.
// hack to redirect to a section page
const menuLinks = document.querySelectorAll(".md-nav--primary .md-nav__list li.md-nav__item--nested");
menuLinks.forEach(function(menuLink) {
menuLink.addEventListener('click', function(event){
const linkName = event.target.parentElement.childNodes[3].innerText.toLowerCase();
let newLink = window.location.origin + "/"
if (window.location.pathname.indexOf("/2021/") === -1){
newLink += linkName;
} else {
// if we are on fabcloud's domain
newLink += "2021/diane.wakim/" + linkName;
}
window.location.href = newLink;
});
},
);
This code is located in docs/javascript/extra.js
, and this file is then added to the configuration file in the same way than the CSS file.
Media content
Images
In order to upload only small images (due to the size limitation on the repo, but also because they will load faster!), I used ImageMagick to resize them. ImageMagick is a command-line tool to edit or convert images, used in the following way:
$ convert avatar-diane.jpg -resize 400x400 avatar-diane.jpg
When I needed to bundle some pictures together, I used the online tool Photocollage.
When writing your Markdown code, be careful with the path of your images! This is one of the most common errors if you added an image to your page and cannot see it. To understand how this works, you have to see the folders of the repository as a tree structure:
A tree visualisation of some folders and files of the repository
Let's say I want to display the image image1.jpg
, which I added in the images folder, in two different pages:
- The homepage of the site, which content is in
docs/index.md
; - The second week assignment page, which content is in
docs/assignments/week02.md
.
From the homepage, the images folder is on the same level, so I can just write the following path to the image, going into the images "branch":

From the assignment page, I'm not on the same level anymore: I'm now in the assignments folder. So I need to go back on the previous branch of the tree so I can go in the images folder. We write this "going back" with two dots like this : ".."

GIFs
I used a lot of GIFs and videos in my documentation, but the space being limited, I mostly uploaded them to different platforms.
To create GIFs, I usually use ezGIF. I then upload them to Giphy, and insert them in the code like an external image:
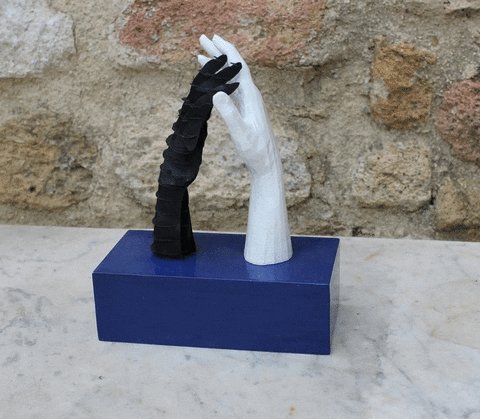
Videos
If I need to edit a video, I use the software VSDC, which is free and has a lot of features. I then upload them to YouTube and embed them in the page with the embed code given by YouTube:
<iframe width="560" height="315" src="https://www.youtube.com/embed/OMnRZfeHFGY" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
Code
When I added code excerpts to my documentation, I tried to render in the most readable way. In Markdown, you can use the color schemes of most languages by adding the name of the language in front of the backticks used to create a block of code. For example, to get the above HTML example of the video iframe, it would look like this:
Emojis
I love emojis, I put them everywhere (did you notice? 🤭). I copy/paste them from Emojipedia.